Python call async from sync
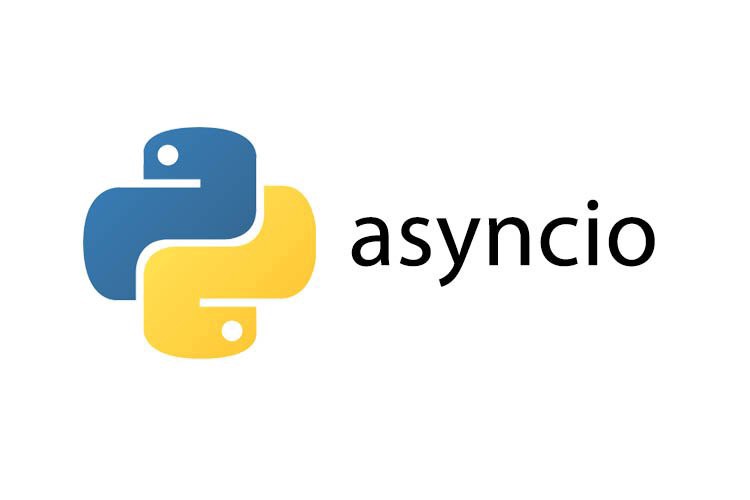
There is a known issue in Python - you have to choose between sync and async code models.
And if you are using async code you can call sync code but from that code you CAN’T call async code again.
Why does this problem occur? The event loop used by the async code is already stuck waiting for the result from the sync code. And if you want to call async code now, you cannot reuse the same event loop.
It looks like an architecture design failure.
But we found workaround for this: https://github.com/erdewit/nest_asyncio
It works just fine!
import asyncio
import nest_asyncio
nest_asyncio.apply()
# Assume this is a very complex async function that you don't want to make sync
async def your_complex_async_function():
return 'result from async function'
def sync_wrapper_which_calls_async_func():
loop = asyncio.get_event_loop()
coroutine = your_complex_async_function()
result = loop.run_until_complete(coroutine)
return result
# Assume this is a library function which make callbacks and you want it to callback your async function
def library_sync_function(sync_callback):
result = sync_callback()
result += ', called from sync function'
return result
async def async_main_function():
result = library_sync_function(sync_wrapper_which_calls_async_func)
result += ', called from async program'
print(result)
return
if __name__ == "__main__":
loop = asyncio.get_event_loop()
loop.run_until_complete(async_main_function())
loop.close()